Computer Graphics Exercises [2/9]
Computer Graphics Exercises
This program draws four discs lying on a diagonal line. For all given discs, the distance to the top of the screen equals the distance to the left edge of the screen. This implies that the coordinates x and y of disc centers are identical for any given disc.
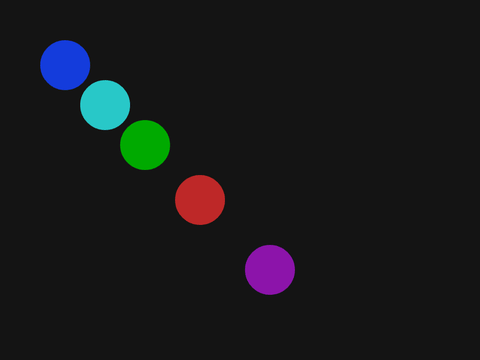
Exercise 1:
Draw a cyan disc somewhere on a line between the blue and the green disc.
Solution
disc(210, 210, 50, /cyan);
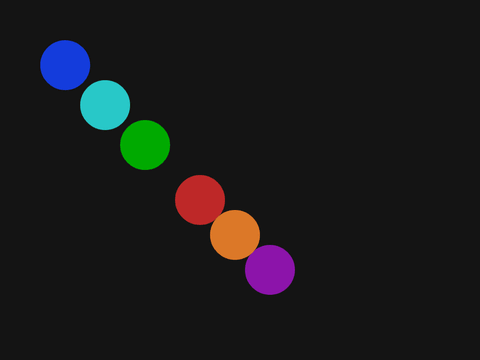
Exercise 2:
Draw an orange disc somewhere on a line between the red and the purple disc.
Solution
disc(470, 470, 50, /orange);
This program draws a horizontal line of discs at the top of the screen. Disc centers are 50 pixels apart and 15 pixels away from the top screen edge. Disc radius is 10 pixels.
Exercise:
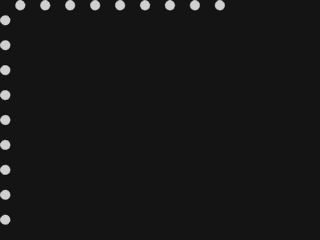
Make another line of discs, but this one should be vertical. Disc centers should be 15 pixels away from the left screen edge.
Do not add another for loop, use just the given one. Make the loop draw another disc in each iteration, in addition to the disc drawn on top of the screen.
The disc function has the form:
disc(x, y, radius)
Hint
Which coordinate, x or y, increases downwards?
The solution is on the next slide.
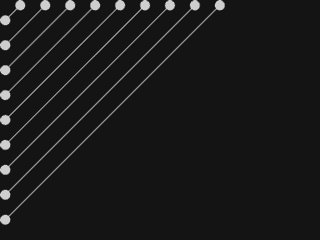
Connect each pair of discs by a line. Do not add another for loop, use just the given one.
Each line should span from one disc center to the other disc center.
The line function has the form:
line(x1, y1, x2, y2)
Solution
for #i=40..720 +=50 { disc(i, 15, 10); disc(15, i, 10); line(i, 15, 15, i); sleepMs(100); }
(Note: the solution with line endpoints swapped is also correct.)
The given program draws discs positioned on a vertical line at x = 480. Disc centers are 50 pixels apart.
Some exercises must be difficult. If all of them could just be easily solved by everyone, then the exercises would be boring, and they would lack educational value. A lot can be learned from a difficult exercise, escpecially when the solution is peeked at only after a few minutes of sincere effort.
Exercise:
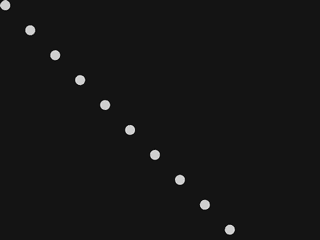
Change the program so that discs are drawn on a diagonal line. The distance from the center of any disc to the top screen edge equals the distance to the left screen edge, which means that the x and y coordinates of disc centers must be equal ( x = y ).
Hint 1
The task requires changing the position of the discs on the left-right axis. Therefore, the x-coordinate of the discs needs to be modified.
Hint 2
The first argument of the disc function specifies the x-coordinate. Also try re-reading the exercise text.
The solution is on the next slide.
[Run the program]
Exercise:
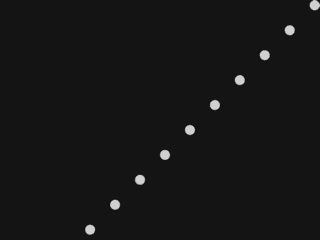
Make another line of discs, but this time on the other diagonal of the screen. The screen is 960 pixels wide.
Hint 1
The only thing that needs changing is the first argument of the disc function call.
Hint 2
A subtraction operation needs to be used for calculating the coordinates, because subtraction can reverse a direction.
The solution is on the next slide.
[Run the program]
Exercise:
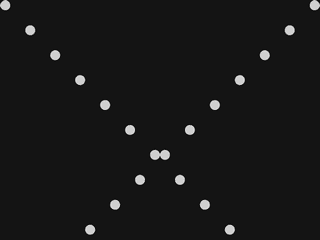
Make the program draw discs on both diagonals. Use only one for loop. Draw two discs in each iteration of the for loop, one disc on each side of the screen.
The solution is on the next slide.
[Run the program]
Exercise:
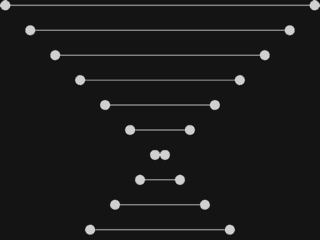
Connect each horizontal pair of discs with a horizontal line. Each line should span from one disc center to the other disc center.
Hint 1
Since lines connect disc centers, the line function uses the same coordinates as the disc functions.
Solution
for #i=10..720 +=50 { disc(i, i, 10); disc(960-i, i, 10); line(i, i, 960-i, i); sleepMs(100); }
This program draws horizontal lines. The lines are 50 pixels apart. One end of each line is at x=100, and another is at x=800.
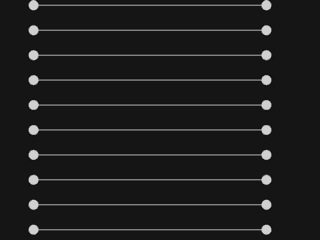
Make the program draw discs at line ends. Disc radius must be 10 pixels. All discs must be drawn before any line is drawn.
To accomplish this task, write another for loop before the given one. The loop should draw two discs on each iteration.
The solution is on the next slide.
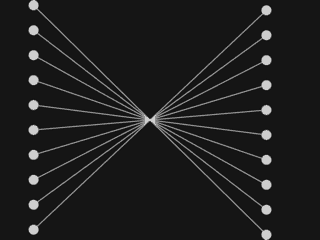
Change the program so that the right column of discs is drawn in the opposite order, from the bottom to the top. The screen is 720 pixels high.
Then, change the second for loop so that the corresponding discs are connected by lines.
Hint 1
It is easier to make changes in the first for loop. When it works correctly, make changes in the second for loop.
Hint 2
Since this task requires a reversal of direction, a subtraction operation needs to be used.
Hint 3
Which coordinate needs changing, x or y?
Solution
for #i=10..720 +=50 { disc(100, i, 10); disc(800, 720-i, 10); sleepMs(100); } for #i=10..720 +=50 { line(100, i, 800, 720-i); sleepMs(100); }
Click on the 'Articles' button at the top to select another article. We also suggest taking a peak into our main 'Gallery' of programs, and taking a look into 'Overview' of our main programming tutorial for beginners. Have fun!
<< F2:Prev - - F4:Next >>