The if Statement [3/16]
The if Statement
The if statement executes the following statement block only if the given condition is true. The if statement in the given program uses a condition x>5.
After you run the program, click on the "Restart" button several times to observe the behavior of the program.
An if statement can optionally have the else part.
After you run the program, click on the "Restart" button several times to observe the behavior of the program.
This program rolls the dice 19 times and notifies you if it gets a 6.
[Run the program]
The print statement is the same as println, except that it doesn't necessarily advance to the next line.
Exercise:
Modify the program such that it outputs a notification when the dice rolls a number smaller than 3.
This program rolls two dice 19 times and notifies you if it gets two equal numbers.
[Run the program]
Exercise:
Modify the program such that it also outputs the sum of the two rolled numbers.
[Run the program]
This program draws 50 discs. The smaller ones are painted red, and the bigger ones are in blue.
Exercise:
Modify this program such that the discs on the left half of the screen are painted in red, and the ones on the right half of the screen are in blue.
If you can't solve this exercise, take a peek at the solution. You don't need to solve every exercise by yourself. A lot can be learned by simply reading the solution and applying it.
Solution
if x<480 : mycolor = /red;
(Note: change the highlighted expression, nothing else.)
This program draws 150 discs. The discs in the left third of the screen are painted in red, the discs in the right third of the screen in orange, and the ones in the middle are in blue.
Exercise:
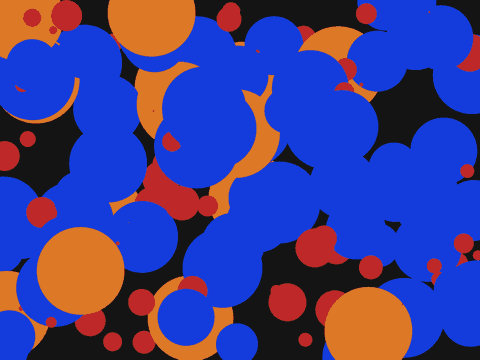
Modify this program such that the discs with a radius smaller than 40 are painted in red, the discs with a radius greater than 85 are painted in orange, and the other ones are in blue.
Solution
if radius<40 : mycolor = /red; if radius>85 : mycolor = /orange;
To check whether a number is within a given range, two conditions must be used.
The conditions can be joined by the operator and.
This example contains an error. The given syntax cannot be used to check if x is between 4 and 7.
Correct this example by using the and operator to join two conditions, as in the previous example.
Run the program to verify that it works correctly.
If there are multiple conditions, they can be separated by commas (,) instead of using the operator and.
This feature is available only in ZedLX. Other programming languages require the use of the operator and.
The operator or can be used to join two conditions.
This program draws 150 discs. The discs in the middle of the screen are painted in red, and the discs on the sides are in blue.
Exercise:
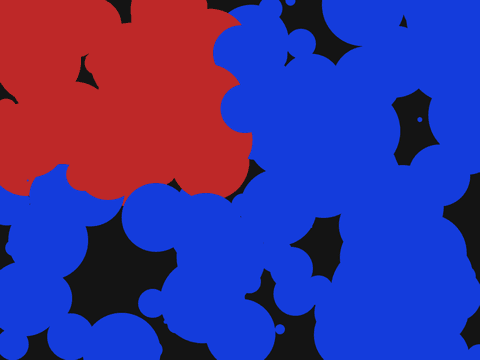
Modify this program such that only the discs in the top left quarter of the screen are painted in red, and other ones in blue.
Solution
if x<480 and y<360 : mycolor = /red;
This program draws 150 discs. The discs in the upper third and bottom third of the screen are painted in red, and the ones in the middle are in blue.
Exercise:
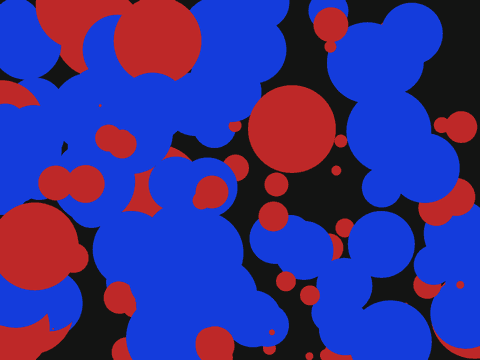
Modify this program such that only the discs with a radius smaller then 40 or radius greater than 85 are painted in red, and other ones in blue.
Solution
if radius<40 or radius>85 : mycolor = /red;
This program draws 2000 small discs. The discs to the right of line x=300 and above line y = 500 are painted in red, and the other ones are in blue.
Exercise:
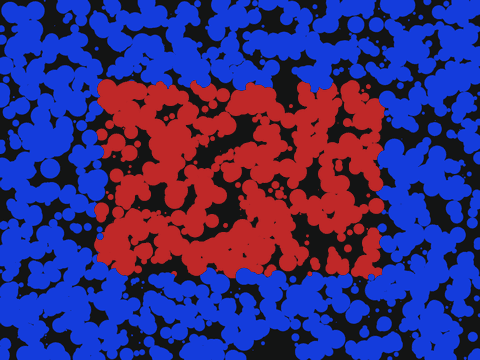
Imagine a rectangle in the middle of the screen with the left edge at x = 200 , right edge at x = 760 , top edge at y = 170 and bottom edge at y = 550 . Modify the given program such that only the discs whose centers lie in the descibed rectangle are painted in red, and other discs in blue.
Solution
if x>200, x<760, y>170, y<550 : mycolor = /red;
Some of the comparison operators include:
< | less than |
> | more than |
<= | less than or equal to |
>= | more than or equal to |
= | equal to |
<> | not equal to |
This program uses several if statements to draw a row of discs in various colors.
The else-if chain can be used to accomplish the same result.
<< F2:Prev - - F4:Next >>