How to Draw N-sided Regular Polygons [1/11]
How to Draw Regular Polygons
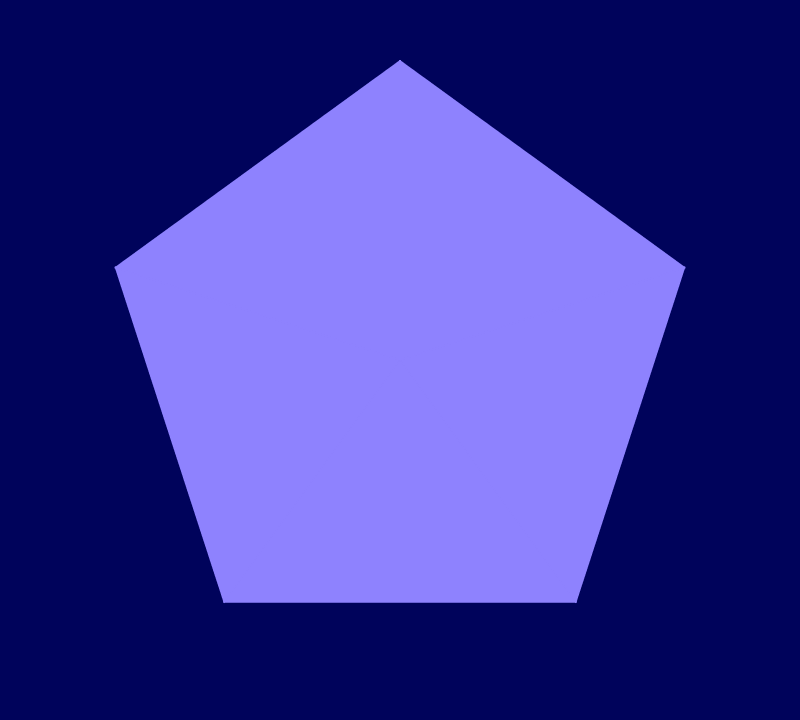
This tutorial explains how to draw regular polygons with a computer program. This is a detailed step-by-step guide, featuring many examples. The provided explanations are valid for drawing regular polygons in any programming language.
Start
To start simple, the provided program only draws one red disc and one green arrow.
[Run the program by clicking the 'Run' button]
The first statement of the program sets the center of the red disc to be at coordinates 480 , 360 , which are at the center of the output image. A red disc of radius 20 is then drawn to visualize the center point.
The sleepMs function then makes a small delay,
so that the red center point can be easily observed.
[Run the program by clicking the 'Run' button]
The Vector Up
The next statement sets the variable size to equal 200 pixels. Then the vector v is created, of length equal to size.
The vector v is then visualized in the green color
by the function DrawArrow . Surprisingly,
the vector v has a direction up.
In 2D computer graphics, the y coordinate is always reversed upside-down,
meaning that the y coordinate increases downwards.
[Run the program by clicking the 'Run' button]
[Click on the 'Next' button (on the page top) to read the next page, or press the F4 key]
2. Drawing a Regular Triangle
The provided program draws the simplest regular polygon: a triangle.
[Run the program by clicking the 'Run' button]
Most parts of the provided source code are not necessary for just drawing a triangle. Those parts were added as a visual assistance for this tutorial.
The Top Vertice
In this section of the program, a point named p1 is created.
This point is the top vertice od the drawn triangle.
The position of the point p1 is calculated in the program by
expression center + v, which adds the green vector v
to the center point.
The point p1 is then visualized in the olive color.
[Run the program by clicking the 'Run' button]
[Click on the 'Next' button (on the page top) to read the next page, or press the F4 key]
3. Calculating The Left Vertice
The next step of generating the regular triangle is to draw the left vertice.
The left vertice has to be placed in a precise position, so
the position of the vertice needs to be computed first.
[Run the program by clicking the 'Run' button]
The simplest way to corectly compute the position of the left vertice is to
perform a vector rotation. Compared to the previous page,
the green vector has been rotated by 120 degrees counter-clockwise.
[Run the program]
Rotations of Vectors
The vector named v2 is introduced in the source code as equal to the vector v rotated by 120 degrees counter-clockwise. The rotation in degrees is performed by the member function rotdg. In 2D computer graphics, since the y coordinate is always reversed upside-down, so is the direction of rotations.
In the next statement, the vector v2
is visualized by a green arrow. Then a slight delay is made so that the green
arrow can be easily observed.
[Run the program]
The Azure Vertice
The next statement creates the left vertice named p2 , and puts it at the position computed by adding the green vector v2 to the center point. The point p2 is then visualized in the azure color.
On the next page, the source code gets greatly simplified.
4. Simplifying the Drawing of the Regular Triangle
On this page, the drawing procedure is much simpler.
All three vertices p1
, p2 , p3
are now computed together in three consecutive statements.
Then, those vertices are visualized by discs in the olive, azure and purple colors.
[Run the program by clicking the 'Run' button]
The center point still needs to exist,
but there is no need to visualize the center point anymore.
On the previous page, the center point was visualized in red,
but now the visualization of the center point has been removed.
[Run the program by clicking the 'Run' button]
In the last statement of the source code, the call to the function trianglep is performed. It draws the blue triangle. The first three arguments of the function call are the three vertices. The last, fourth argument is the blue color.
If you like this tutorial, you can use the gray sharing buttons to share it with your friends. The sharing buttons create a link to the current page. The buttons for Twitter, Facebook, Pinterest, Reddit and LinkedIn are provided.
On the next page, the regular triangle is generated by using iteration.
5. Iterating the Rotations
On this page, a three-pronged star is generated by iteration. Drawing by iteration is an important sub-step in the quest of drawing regular polygons with any number of sides.
The iteration is performed by the for loop.
The number of iterations is 3.
[Run the program by clicking the 'Run' button]
The loop variable i
contains the ordinal number of the current iteration.
This variable is printed out on each iteration by the first
println statement.
[Run the program]
In each iteration, a point named pA is computed. The point pA is then visualized as the red vertice.
A yellow line is drawn next. One endpoint of the line is the point pA and the other is the point center. The line is drawn by the function linewp, with a width of 5 pixels. Width of the line is the third argument of this function.
The final statemt in the body of the for loop is very important. It rotates the vector v by 120 degrees counter-clockwise. The new value of the rotated vector v is then employed in the next iteration, to draw the next vertice and the next side of the regular triangle.
On the next page, we draw a regular pentagon by iteration.
6. Drawing the Regular Pentagon
On this page, the regular pentagon is generated by iteration.
The first statement of the source code sets the number of
sides n to equal 5.
In the first statement, the type int indicates
that the number of sides must be a whole number.
[Run the program by clicking the 'Run' button]
The fourth statemet of the program computes the rotation angle, which must equal the full circle of 360 degrees divided by the number of sides.
A very important change is that the for
loop now has exactly n iterations.
For the regular pentagon, the number of iterations is 5.
[Run the program]
In each iteration, two points named pA and pB are computed. The point pA is immediately visualized as the red vertice.
On the previous page, the angle of 120 degrees was employed to draw the regular triangle. Now, the computed value named angle is the argument of both rotations that previously used the angle of 120 degrees.
The point pB is not immediately visualized. Instead, this point is employed as the endpoint of a yellow line that visualizes one side of the regular triangle.
The final statemt in the body of the for loop is rotates the vector v counter-clockwise, by the computed angle in degrees.
On the next page, the drawings of n-sided regular polygons are further improved.
7. Drawing a Random Regular Polygon
The first statement of the source code sets the colors to dark blue and gold.
The clearScr function allows a default background color and a default drawing color to be provided.
It enables both colors to be specified in a single line of the source code.
In the source code of this program, other statements do not specify explicit colors anymore,
which causes the default drawing colors to be used.
[Run the program]
The Random Number of Sides
The function rn with an argument of 10 returns a random number
in range of 1 - 10. The constant 2 is added to that random value, so that the number of sides
n will equal a random number in range 3-12.
[Run the program]
The body of the for loop has been modified so that
the random regular polygon is filled with color.
[Run the program]
On the next page, the regular star septagon is generated.
8. Drawing the Regular Star Polygons
To draw the regular star septagon, the number of sides n
must be set to 7. An additional variable is required, named skip,
which tells how many vertices to skip over during iteration.
[Run the program]
The computation of the angle has been modified compared to the previous page. The value skip is now employed to compute the angle.
No other major changes are required to draw the regular star septagon.
[Run the program]
If you like this tutorial, you can use the gray sharing buttons to share it with your friends. The sharing buttons create a link to the current page. The buttons for Twitter, Facebook, Pinterest, Reddit and LinkedIn are provided.
The next page adds a simple animation.
9. Animating Regular Polygons
The given program creates a simple animation of a moving regular polygon.
[Run the program]
The regular polygon is generated by the user-defined function DrawPolygon. This function is called from the last statement in the while loop.
The function DrawPolygon has three parameters.
The first parameter is the number of sides. The second parameter is the position.
The third parameter is the radius of the polygon in pixels.
[Run the program]
The usual facilities for animating objects in ZedLX have been employed. It is very easy to add animation to ZedLX programs. The source code shows the facilities employed to create this animation: vertical synchronization by vsync, clear screen, and the current time.
On the next page, the polygon can be moved by mouse.
10. Following the Mouse Motion
The polygon is now following the mouse pointer.
[Run the program]
To create this interactive demo, the usual facilities for animating objects in ZedLX have been employed. The value of elapsedTime has been computed in the usual maner, as it is descibed in the ZedLX tutorial chapter "Time and Animation".
The vector difference is the difference between the current mouse position and the center of the polygon. To make the polygon move slowly, the position of the polygon is changed in small steps. The position is held in the variable named position. The position is moved in each frame by only a small fraction of the difference.
On the next page, the polygon can be moved by the keyboard keys.
11. Moving the Regular Polygon by Keyboard
The polygon can now be moved by the arrow keys.
[Run the program]
The vector velocity represents the polygon's speed and direction. The velocity is modified by pressing the arrow keys.
On each display frame, the position of the polygon is updated according
to the rule of motion vectors. The rule is implemented in the penultimate statement of the
while loop:
posNew = posOld + v×t;
In the equation above, posNew is a new position,
posOld is an old position,
v is a vector of velocity,
and t is the elapsed time.
With this page, we complete our tutorial on drawing the regular polygons. Click on the 'Articles' button at the top to select another article. We also suggest taking a peak into our main 'Gallery' of programs, and taking a look into 'Overview' of our main programming tutorial for beginners. Have fun!
<< F2:Prev - - F4:Next >>